接口
# Go 接口介绍
在 Go 语言中,**接口(interface)**是一种抽象类型,定义了一组方法的集合。接口指定了这些方法的签名,而不关心具体的实现。任何类型只要实现了接口中定义的所有方法,就可以被视为实现了该接口。
接口是实现多态和灵活性的重要工具,可以让代码更具通用性、解耦性和模块化。
# 1. 接口的定义
接口定义一个方法的集合,语法如下:
type InterfaceName interface {
Method1(param1 Type1, param2 Type2) ReturnType
Method2() ReturnType
}
1
2
3
4
2
3
4
示例:
type Shape interface {
Area() float64
Perimeter() float64
}
1
2
3
4
2
3
4
在上面,Shape
接口定义了两个方法 Area
和 Perimeter
,任何类型只要实现了这两个方法,就实现了 Shape
接口。
# 2. 类型实现接口
在 Go 中,类型实现接口的方式是自动的,无需显式声明。例如:
type Circle struct {
Radius float64
}
func (c Circle) Area() float64 {
return 3.14 * c.Radius * c.Radius
}
func (c Circle) Perimeter() float64 {
return 2 * 3.14 * c.Radius
}
// Circle 实现了 Shape 接口
1
2
3
4
5
6
7
8
9
10
11
12
13
2
3
4
5
6
7
8
9
10
11
12
13
# 3. 使用接口
我们可以通过接口实现多态:
func PrintShapeInfo(s Shape) {
fmt.Printf("Area: %f\n", s.Area())
fmt.Printf("Perimeter: %f\n", s.Perimeter())
}
func main() {
c := Circle{Radius: 5}
PrintShapeInfo(c)
}
1
2
3
4
5
6
7
8
9
2
3
4
5
6
7
8
9
运行结果:
Area: 78.500000
Perimeter: 31.400000
1
2
2
# 4. 空接口(interface{}
)
空接口是 Go 中一个特殊的接口,它不包含任何方法,因此所有类型都实现了空接口。这使得空接口可以用来表示任意类型。
# 空接口的使用场景
- 存储任意类型的数据:
func PrintAnything(value interface{}) {
fmt.Printf("Value: %v, Type: %T\n", value, value)
}
func main() {
PrintAnything(42) // 输出: Value: 42, Type: int
PrintAnything("Hello") // 输出: Value: Hello, Type: string
PrintAnything(3.14) // 输出: Value: 3.14, Type: float64
}
1
2
3
4
5
6
7
8
9
2
3
4
5
6
7
8
9
- 实现泛型容器:
type Container struct {
items []interface{}
}
func (c *Container) Add(item interface{}) {
c.items = append(c.items, item)
}
func (c *Container) Get(index int) interface{} {
return c.items[index]
}
func main() {
c := &Container{}
c.Add(42)
c.Add("Hello")
c.Add(3.14)
fmt.Println(c.Get(0)) // 输出: 42
fmt.Println(c.Get(1)) // 输出: Hello
fmt.Println(c.Get(2)) // 输出: 3.14
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
# 5. 类型断言
在使用接口时,如果需要将接口转换为具体类型,可以使用类型断言。
func main() {
var i interface{} = "Hello"
// 类型断言
str, ok := i.(string)
if ok {
fmt.Println("String value:", str) // 输出: String value: Hello
} else {
fmt.Println("Not a string")
}
}
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
# 6. 接口组合
Go 支持接口的组合,通过将多个接口组合成一个新的接口。
type Reader interface {
Read(p []byte) (n int, err error)
}
type Writer interface {
Write(p []byte) (n int, err error)
}
type ReadWriter interface {
Reader
Writer
}
1
2
3
4
5
6
7
8
9
10
11
12
2
3
4
5
6
7
8
9
10
11
12
ReadWriter
接口组合了 Reader
和 Writer
的方法,任何同时实现了 Read
和 Write
方法的类型都实现了 ReadWriter
接口。
# 7. 接口的实际案例
# 7.1 文件操作
Go 标准库中的 io
包定义了一些常用的接口,例如 Reader
、Writer
等。
package main
import (
"fmt"
"io"
"os"
)
func CopyContent(r io.Reader, w io.Writer) {
_, err := io.Copy(w, r)
if err != nil {
fmt.Println("Error copying content:", err)
}
}
func main() {
file, err := os.Open("input.txt")
if err != nil {
fmt.Println("Error opening file:", err)
return
}
defer file.Close()
CopyContent(file, os.Stdout)
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
# 7.2 HTTP 处理
Go 的 net/http
包中使用接口来处理 HTTP 请求,例如 http.Handler
接口:
package main
import (
"fmt"
"net/http"
)
type MyHandler struct{}
func (h MyHandler) ServeHTTP(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, this is a custom handler!")
}
func main() {
handler := MyHandler{}
http.ListenAndServe(":8080", handler)
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
# 7.3 数据库操作
Go 的 database/sql
包中使用接口定义了数据库操作,例如 sql.DB
使用了 Driver
接口。
# 8. 接口的优缺点
优点:
- 灵活性:接口允许在不同类型之间共享行为,实现多态。
- 解耦性:使用接口可以减少模块之间的依赖。
- 可扩展性:接口可以让代码更加模块化,易于扩展。
缺点:
- 运行时错误:使用类型断言时,如果类型不匹配,会导致运行时错误。
- 可读性:过多使用空接口会降低代码的可读性和类型安全性。
# 总结
- 接口是 Go 语言中实现多态和灵活性的重要工具。
- 空接口
interface{}
可以表示任意类型,但使用时需小心类型断言。 - 接口组合允许通过组合多个接口实现更复杂的功能。
- 接口在实际项目中被广泛用于文件操作、HTTP 服务、数据库操作等场景。
掌握接口的用法是学习 Go 的关键步骤,有助于编写高效、灵活的代码。
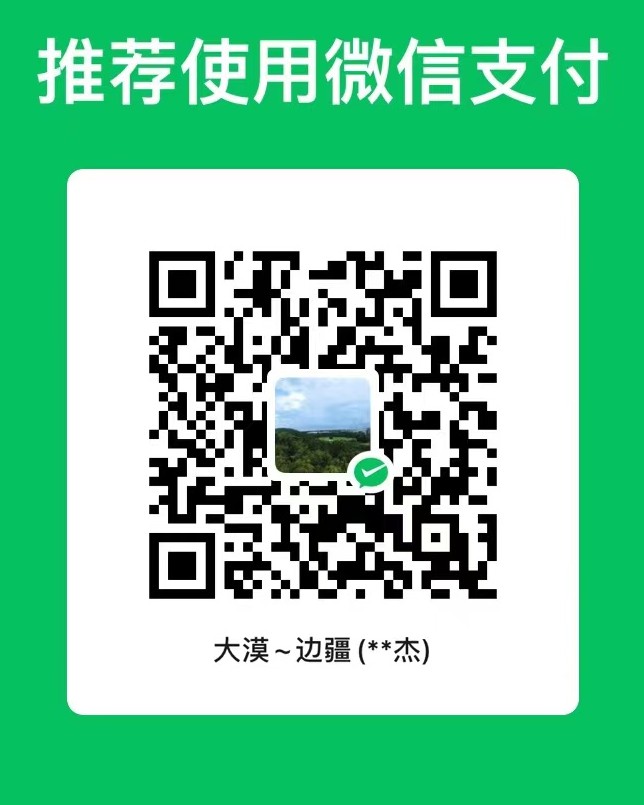
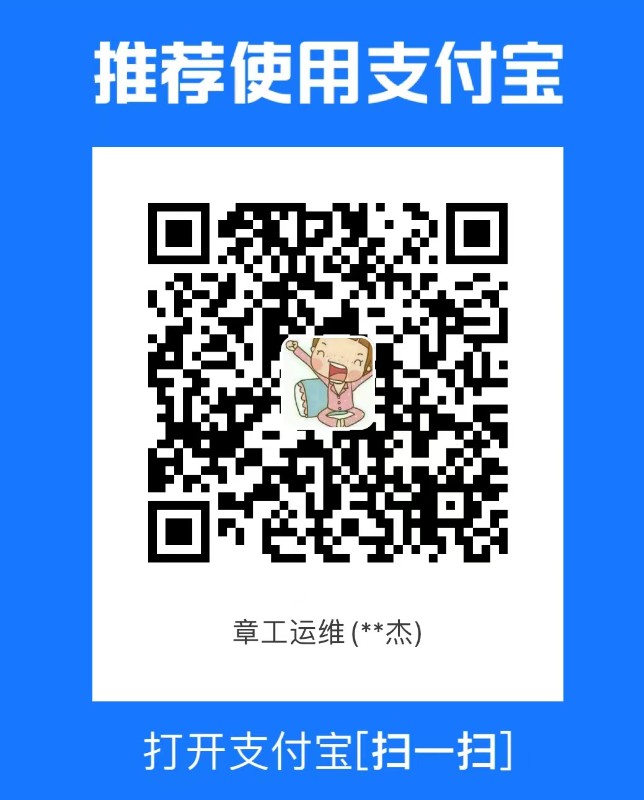
上次更新: 2024/11/21, 10:50:23