使用go和vue编写学生管理系统
# yarn安装vue3项目
全局安装yarn
npm install yarn -g
切换源 yarn config get registry npm config set registry https://registry.npmjs.org/
安装新版: yarn global add @vue/cli 检查vue是否被安装 vue -V 创建项目(与npm创建vue3项目一样) vue create my-project 我们一般使用第一条(默认)、vuex、router,css预处理,ts等/完成之后,进入到创建的项目根目录下 cd my-project 安装依赖包 yarn 说明:yarn是yarn install的简写,可直接敲击yarn,功能和npm install一样 最后运行项目 yarn run serve 浏览器会自动打开运行一个页面,出现以下页面,说明成功了。
src/App.vue文件
<template>
<!-- <img alt="Vue logo" src="./assets/logo.png">-->
<!-- <HelloWorld msg="Welcome to Your Vue.js App"/>-->
<div id="app">
<StudentManager />
</div>
</template>
<script>
// import HelloWorld from './components/HelloWorld.vue'
import StudentManager from './components/StudentManager.vue';
export default {
name: 'App',
components: {
StudentManager
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
src/components/StudentManager.vue文件内容
<template>
<div>
<h1>学生管理</h1>
<form @submit.prevent="addStudent">
<input v-model="newStudent.name" placeholder="姓名" required />
<input v-model="newStudent.age" type="number" placeholder="年龄" required />
<input v-model="newStudent.grade" placeholder="年级" required />
<button type="submit">添加学生</button>
</form>
<ul>
<li v-for="student in students" :key="student.id">
{{ student.name }} - {{ student.age }} - {{ student.grade }}
<button @click="editStudent(student)">编辑</button>
<button @click="deleteStudent(student.id)">删除</button>
</li>
</ul>
<div v-if="editingStudent">
<h2>编辑学生</h2>
<form @submit.prevent="updateStudent">
<input v-model="editingStudent.name" placeholder="姓名" required />
<input v-model="editingStudent.age" type="number" placeholder="年龄" required />
<input v-model="editingStudent.grade" placeholder="年级" required />
<button type="submit">更新学生</button>
</form>
</div>
</div>
</template>
<script>
export default {
data() {
return {
students: [],
newStudent: {
name: '',
age: '',
grade: ''
},
editingStudent: null
};
},
methods: {
fetchStudents() {
fetch('http://localhost:8001/students')
.then(response => response.json())
.then(data => {
this.students = data;
});
},
addStudent() {
fetch('http://localhost:8001/students', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(this.newStudent)
})
.then(response => response.json())
.then(data => {
this.students.push(data);
this.newStudent = { name: '', age: '', grade: '' };
});
},
editStudent(student) {
this.editingStudent = { ...student };
},
updateStudent() {
fetch(`http://localhost:8001/students/${this.editingStudent.id}`, {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(this.editingStudent)
})
.then(response => response.json())
.then(data => {
const index = this.students.findIndex(s => s.id === data.id);
this.students.splice(index, 1, data);
this.editingStudent = null;
});
},
deleteStudent(id) {
fetch(`http://localhost:8001/students/${id}`, {
method: 'DELETE'
})
.then(() => {
this.students = this.students.filter(student => student.id !== id);
});
}
},
mounted() {
this.fetchStudents();
}
};
</script>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
# 创建go项目
1.创建项目目录
mkdir student-management
cd student-management
go mod init student-management
1
2
3
2
3
2.安装gorilla/mux:
go get -u github.com/gorilla/mux
1
3.主文件程序
package main
import (
"encoding/json"
"log"
"net/http"
"strconv"
"github.com/gorilla/handlers"
"github.com/gorilla/mux"
)
type Student struct {
ID int `json:"id"`
Name string `json:"name"`
Age int `json:"age"`
Grade string `json:"grade"`
}
var students []Student
var nextID = 1
func main() {
router := mux.NewRouter()
router.HandleFunc("/students", getStudents).Methods("GET")
router.HandleFunc("/students/{id}", getStudent).Methods("GET")
router.HandleFunc("/students", createStudent).Methods("POST")
router.HandleFunc("/students/{id}", updateStudent).Methods("PUT")
router.HandleFunc("/students/{id}", deleteStudent).Methods("DELETE")
// 添加CORS支持
corsHandler := handlers.CORS(
handlers.AllowedOrigins([]string{"*"}),
handlers.AllowedMethods([]string{"GET", "POST", "PUT", "DELETE"}),
handlers.AllowedHeaders([]string{"Content-Type"}),
)
log.Println("Starting server on :8001")
if err := http.ListenAndServe(":8001", corsHandler(router)); err != nil {
log.Fatalf("Server failed to start: %v", err)
}
}
func getStudents(w http.ResponseWriter, r *http.Request) {
json.NewEncoder(w).Encode(students)
}
func getStudent(w http.ResponseWriter, r *http.Request) {
params := mux.Vars(r)
id, _ := strconv.Atoi(params["id"])
for _, student := range students {
if student.ID == id {
json.NewEncoder(w).Encode(student)
return
}
}
http.NotFound(w, r)
}
func createStudent(w http.ResponseWriter, r *http.Request) {
var student Student
json.NewDecoder(r.Body).Decode(&student)
student.ID = nextID
nextID++
students = append(students, student)
json.NewEncoder(w).Encode(student)
}
func updateStudent(w http.ResponseWriter, r *http.Request) {
params := mux.Vars(r)
id, _ := strconv.Atoi(params["id"])
for i, student := range students {
if student.ID == id {
json.NewDecoder(r.Body).Decode(&student)
student.ID = id
students[i] = student
json.NewEncoder(w).Encode(student)
return
}
}
http.NotFound(w, r)
}
func deleteStudent(w http.ResponseWriter, r *http.Request) {
params := mux.Vars(r)
id, _ := strconv.Atoi(params["id"])
for i, student := range students {
if student.ID == id {
students = append(students[:i], students[i+1:]...)
json.NewEncoder(w).Encode(students)
return
}
}
http.NotFound(w, r)
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
4.启动程序
go run main.go
1
5.跨域问题处理
如果访问前端页面报错
Failed to fetch
TypeError: Failed to fetch
at Proxy.addStudent (webpack-internal:///./node_modules/babel-loader/lib/index.js??clonedRuleSet-40.use[0]!./node_modules/vue-loader/dist/index.js??ruleSet[0].use[0]!./src/components/StudentManager.vue?vue&type=script&lang=js:24:7)
at eval (webpack-internal:///./node_modules/babel-loader/lib/index.js??clonedRuleSet-40.use[0]!./node_modules/vue-loader/dist/templateLoader.js??ruleSet[1].rules[3]!./node_modules/vue-loader/dist/index.js??ruleSet[0].use[0]!./src/components/StudentManager.vue?vue&type=template&id=1c27fbbc:22:137)
at cache.<computed>.cache.<computed> (webpack-internal:///./node_modules/@vue/runtime-dom/dist/runtime-dom.esm-bundler.js:1676:12)
at callWithErrorHandling (webpack-internal:///./node_modules/@vue/runtime-
core/dist/runtime-core.esm-bundler.js:358:19)
at callWithAsyncErrorHandling (webpack-internal:///./node_modules/@vue/runtime-core/dist/runtime-core.esm-bundler.js:365:17)
at HTMLFormElement.invoker (webpack-internal:///./node_modules/@vue/runtime-dom/dist/runtime-dom.esm-bundler.js:883:82)
1
2
3
4
5
6
7
8
9
2
3
4
5
6
7
8
9
解决方法:在go的程序中加入跨域处理
# 页面展示
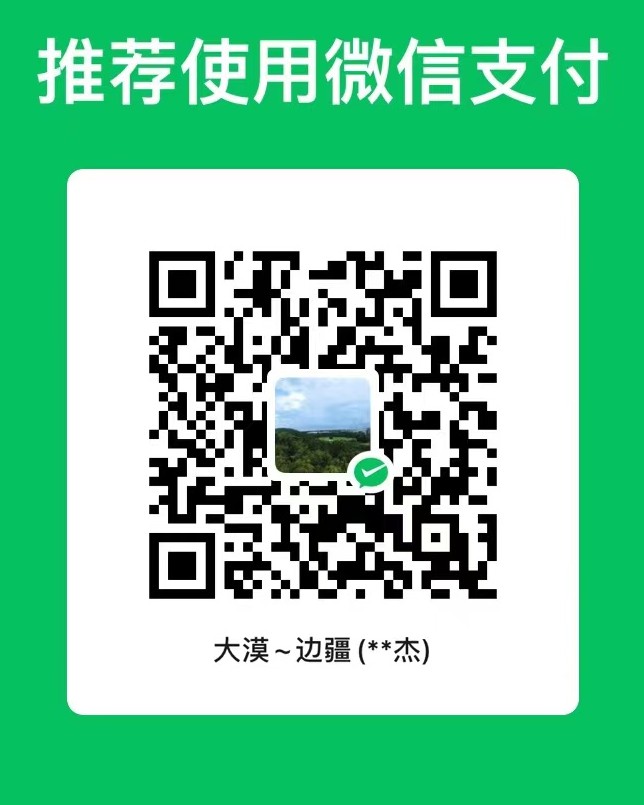
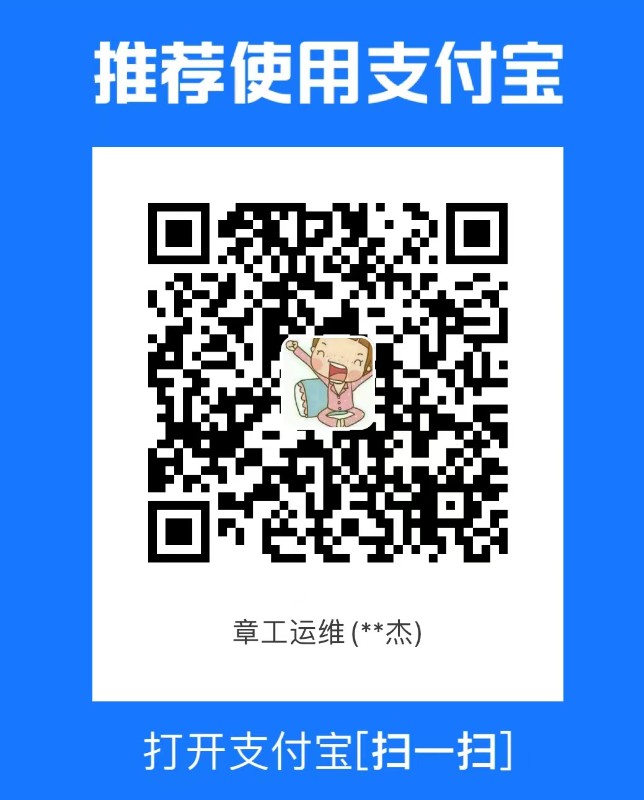
上次更新: 2024/09/01, 20:26:32